The Synchronous and Asynchronous Sides of Javascript
JavaScript is a programming language developed by Netscape Inc. and is often mistaken as java based. It is mainly used for creating highly responsive interfaces that improve user experience(UX) and provide dynamic functionality, without having to wait for the server to react and show a new page. To simply put this, we can say JavaScript is a single-threaded asynchronous execution model that can speed up the entire process and requires comparatively minimum memory when compared to the other languages.
The finest thing about JavaScript is its ability to handle a large number of users. However, there are two different modes synchronous code and asynchronous code. Here, we’ll discuss what sets them apart from each other.
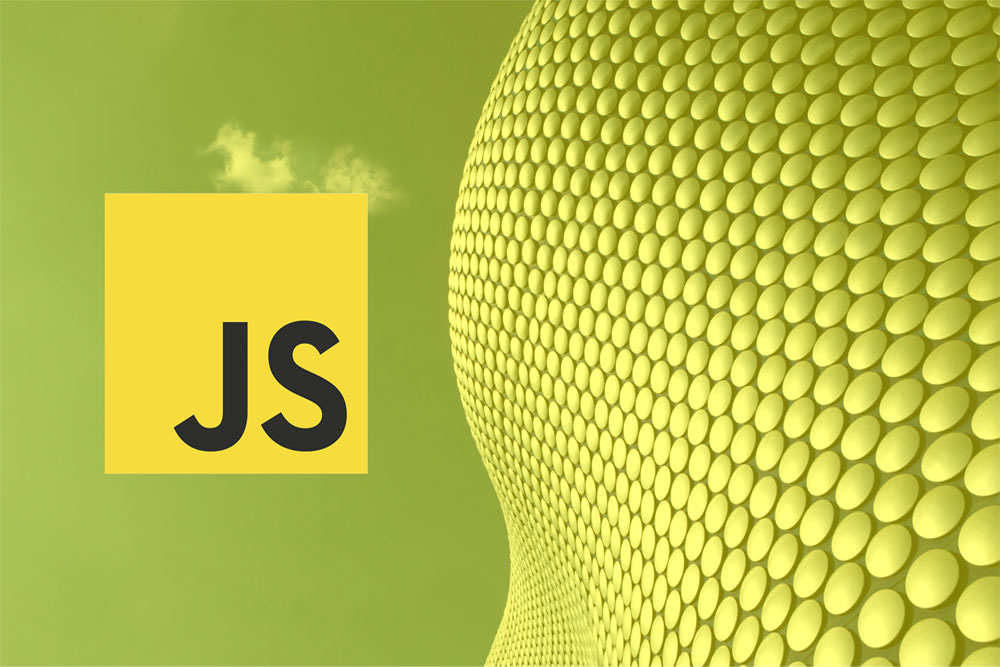
Blocking (Synchronous) code of Node.js for reading files
In blocking code, the processor will execute the instructions sequentially which means the next instruction has to wait until the current instruction is executed. So progressively, the waiting time is high in the buffer pool, at the same time the computers are working efficiently.
For example
const fs = require(‘fs’);
console.log(‘start’);
const data = fs.readFileSync(‘./file.txt’, ‘utf-8’); // blocks here until file is read
console.log(‘data: ‘, data.trim());
console.log(‘end’);
The function “readFileSync” is used to read the file in Synchronous mode or blocking I/O which means that when executing the function “readFileSync”, it will read the file synchronously and in the meantime, the program will wait until the file is completely read. Due to this, the program execution time is relatively high.
start
data: Hello World!
end
Non-Blocking (Asynchronous) code of Node.js for reading files
In non-blocking code, the processor will execute the code in parallel, which means the execution is carried out continuously. It works independently from the outside of the program workflow.
For Example
const fs = require(‘fs’);
console.log(‘start’);
fs.readFile(‘./file.txt’, ‘utf-8’, (err, data) => {
if (err) throw err;
console.log(‘data: ‘, data.trim());
});
console. log(‘end’);
In the asynchronous mode of execution, initially, it prints ‘start’ and then when reading the file using ‘readFile()’ function the next statement is executed without waiting for the completion of the ‘readFile()’ function. Once the file has been read it will execute its callback function to finalize the statement.
start
end
data: Hello World!
What you should consider when making a decision?
- The asynchronous mode of execution is faster than the synchronous model because it won’t wait for the completion of instruction execution.
- Compared to the synchronous, there is a chance to occur error in the asynchronous.
- Working with the asynchronous model is trickier than the synchronous model.
- Asynchronous allows the user to manage the CPU more efficiently than the synchronous model.
Conclusion
To run this shortly, asynchronous has its fair share of benefits and synchronous has its fair share. We hope this helps you understand better what is what and which can you benefit the most from.
Centizen
A Leading IT Staffing, Custom Software and SaaS Product Development company founded in 2003. We offer a wide range of scalable, innovative IT Staffing and Software Development Solutions.
Contact Us
USA: +1 (971) 420-1700
Canada: +1 (971) 420-1700
India: +91 86107-03503
Email: contact@centizen.com
Our Services
Software Development
IT Staffing
General Staffing
Remote Hiring
Products
Contact Us
USA: +1 (971) 420-1700
Canada: +1 (971) 420-1700
India: +91 86107-03503
Email: contact@centizen.com