Mastering Angular TypeScript: Essential Practices for Developers
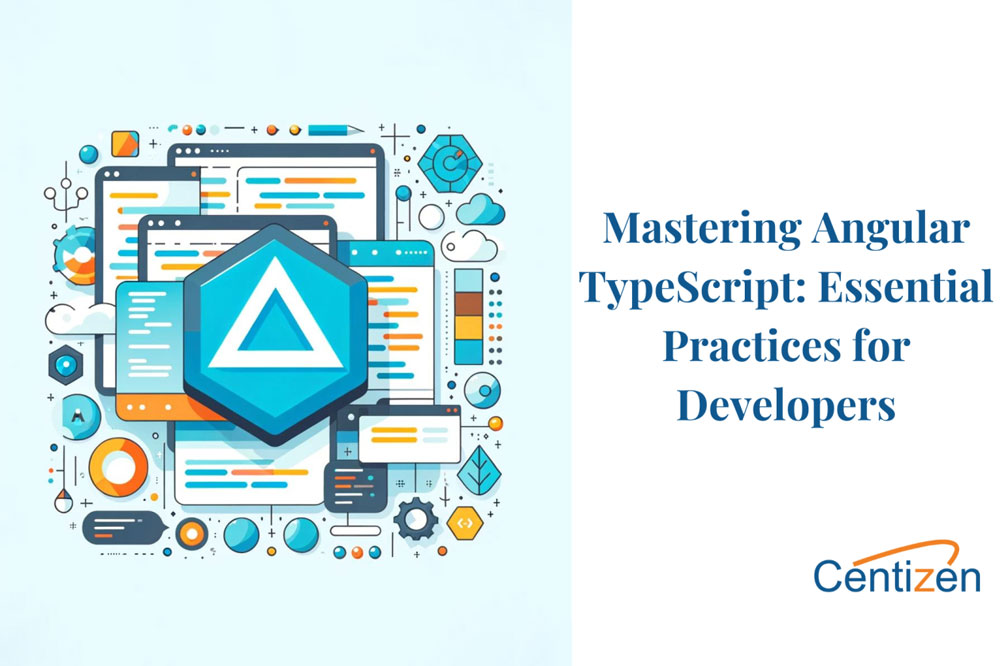
As a developer diving into the world of Angular and TypeScript, adopting the right coding practices is crucial for creating maintainable, efficient, and high-quality applications. Here, we’ll explore a comprehensive set of guidelines to help you improve your coding skills, enhance code readability, and maintain project consistency.
1. Follow Angular style guide
Always refer to the Angular Style Guide for best practices in project structure, naming conventions, and coding practices. This ensures consistency and best practices throughout your project, making it easier for teams to collaborate and maintain the codebase.
2. Component design
Single responsibility: Each component should have a single responsibility and be small and focused. Aim for each file to have fewer than 400 lines of code.
Lifecycle hooks: Use Angular lifecycle hooks (like OnInit, OnDestroy) for related functionality instead of placing all logic in the constructor. This makes your code cleaner and easier to manage.
3. Services and dependency injection
Modular services: Encapsulate business logic in services instead of components. This promotes code reusability and separation of concerns.
Use dependency injection: Utilize Angular’s built-in dependency injection to manage dependencies across your application efficiently.
4. TypeScript specifics
Strong typing: Always declare variables with types unless it is absolutely necessary not to. This helps catch errors during compilation.
Interfaces: Use interfaces for complex data structures passed between components and services. This enhances type safety and code readability.
Access modifiers: Clearly mark the accessibility of class members using public, private, or protected. This improves code maintainability and encapsulation.
5. Reactive programming with RxJS
Unsubscribe observables: Always unsubscribe from observables in the component’s OnDestroy lifecycle hook to prevent memory leaks.
Use operators: Familiarize yourself with RxJS operators like map, filter, mergeMap, etc., to handle asynchronous data effectively.
6. State management
Consider using state management libraries (e.g., NgRx, Akita) if your application complexity grows. This helps in managing state in a predictable way across large applications.
7. Error handling
Implement global error handling using Angular’s ErrorHandler class.
Use interceptors to handle HTTP errors centrally. This ensures a consistent error-handling strategy across your application.
8. Code organization
Feature modules: Organize code into feature modules to encapsulate functionality and promote lazy loading.
Lazy loading: Implement lazy loading for feature modules to reduce the initial load time, improving the performance of your application.
9. Testing
Unit tests: Write unit tests for components and services using Jasmine and Angular Testing Utilities. This ensures that your code works as expected and helps catch bugs early.
E2E tests: Use Protractor for end-to-end testing to ensure the application works as expected from a user’s perspective.
10. Performance optimization
Change detection strategy: Use ChangeDetectionStrategy.OnPush in components where data immutability is maintained to improve performance.
TrackBy function: Use trackBy function in *ngFor to minimize DOM manipulations and enhance performance.
11. Code quality tools
Integrate tools like TSLint and Prettier in your development workflow to ensure code quality and style consistency.
12. Documentation
Comment your code where necessary, especially for public APIs and complex logic. Consider using tools like Compodoc for generating Angular project documentation.
Advanced considerations for continued learning
13. Advanced RxJS patterns
Higher-order observables: Understanding higher-order observables (like switchMap, concatMap, exhaustMap) can be crucial for handling complex asynchronous scenarios.
Custom operators: Create custom RxJS operators if you find yourself repeatedly using the same complex patterns with streams.
14. Optimizing observables
Sharing work with shareReplay: Use shareReplay to avoid unnecessary requests and computations, particularly with HTTP requests.
Avoiding memory leaks: Be mindful of where and how you subscribe to observables. Use patterns like takeUntil to automate unsubscribing.
15. Dynamic component loading
Learn to dynamically load and unload components at runtime using Angular’s ComponentFactoryResolver. This is particularly useful for large applications with modular features that can be loaded on demand.
16. Angular universal
Implement server-side rendering with Angular Universal to improve SEO and optimize the initial load time for your applications.
17. Accessibility
Incorporate accessibility (a11y) from the start: Use semantic HTML, manage focus correctly, and ensure that your application is usable with a keyboard and assistive technologies.
18. Internationalization (i18n)
Use Angular’s internationalization (i18n) tools to prepare your application for localization into multiple languages, which can broaden your user base.
19. Security practices
Sanitization: Use Angular’s DomSanitizer to prevent XSS (Cross-Site Scripting).
HTTP security: Use HTTPS, add CSRF tokens, and manage CORS policies properly to secure your web applications.
20. Advanced Angular CLI usage
Utilize the full power of Angular CLI to automate tasks, enforce architectural decisions, and streamline your development process.
Final thoughts for developers
21. Mentorship and peer review: Engage in peer programming sessions and code reviews with more experienced developers. This not only helps in catching errors and learning best practices but also aids in understanding different approaches to problem-solving.
22. Soft skills development: Work on developing soft skills such as problem-solving, critical thinking, and effective communication. These skills are essential when working in teams, understanding project requirements, and presenting your ideas.
23. Keeping up-to-date: Stay updated with the latest Angular versions and their features. Angular is continuously evolving, and keeping abreast of the changes can ensure you are using the most efficient and powerful features available.
24. Mastering observables beyond RxJS: While RxJS is integral to Angular, understanding other aspects of reactive programming can be beneficial. Explore additional libraries and frameworks that use reactive principles to enhance your versatility in solving programming challenges.
25. Focus on User Experience (UX): Understanding the basics of UX design can significantly impact how you approach front-end development. Knowing how users interact with applications can guide better UI decisions and lead to a more intuitive user interface.
26. Server-side technologies: Gain some knowledge of server-side technologies that commonly interact with Angular applications, such as Node.js and Express for backend services. Understanding the server-side will allow for better decision-making and integration when developing full-stack applications.
27. Learning from failures: Embrace failures and bugs as learning opportunities. Analyzing what went wrong, why it went wrong, and how to prevent it in the future is a critical skill that strengthens your problem-solving capabilities.
28. Documentation and knowledge sharing: Regularly document your learnings and challenges, possibly through blogging or internal wikis. Sharing knowledge not only cements your understanding but can also help your peers.
29. Scalability and architecture: Start to consider how applications can be scaled both in terms of size and complexity. Understanding architectural patterns and how to apply them in Angular can help in designing more robust and scalable applications.
30. Ethics and security: Develop a strong sense of ethical responsibility and prioritize security in your applications from the start. Understanding common security pitfalls and ethical considerations in software development is crucial for building trustworthy applications.
Expanding your expertise with these additional aspects ensures a well-rounded approach to professional development in Angular and software engineering in general. Each theme encourages not just technical growth but also personal and professional development in the field.
By embracing these practices and continually striving to learn and improve, you’ll be well on your way to becoming a proficient and effective Angular developer. Happy coding!
Explore Centizen Inc’s comprehensive staffing solutions, custom software development and innovative software offerings, including ZenBasket and Zenyo, to elevate your business operations and growth.
Centizen
A Leading IT Staffing, Custom Software and SaaS Product Development company founded in 2003. We offer a wide range of scalable, innovative IT Staffing and Software Development Solutions.
Contact Us
USA: +1 (971) 420-1700
Canada: +1 (971) 420-1700
India: +91 63807-80156
Email: contact@centizen.com
Our Services
Products
Contact Us
USA: +1 (971) 420-1700
Canada: +1 (971) 420-1700
India: +91 63807-80156
Email: contact@centizen.com